Below you'll find a list of topics this tutorial covers. The easiest way to work with it is to look at an example, then look at the code shown below the examples.
Basic
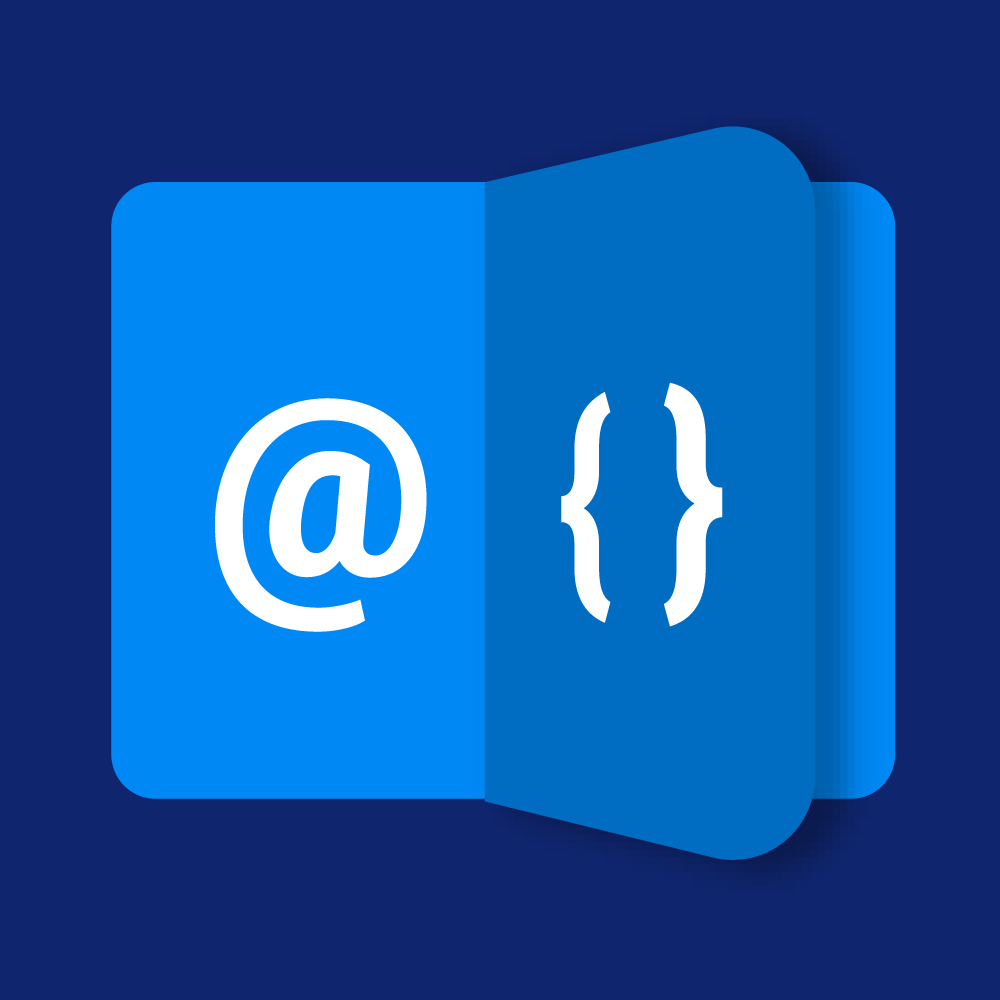
Quick Reference for Razor APIs
Quick Summary of most APIs for reference. This is still WIP...
- Quick Reference for all APIs new v16
2sxc Quick Reference for Razor using the new Typed API.
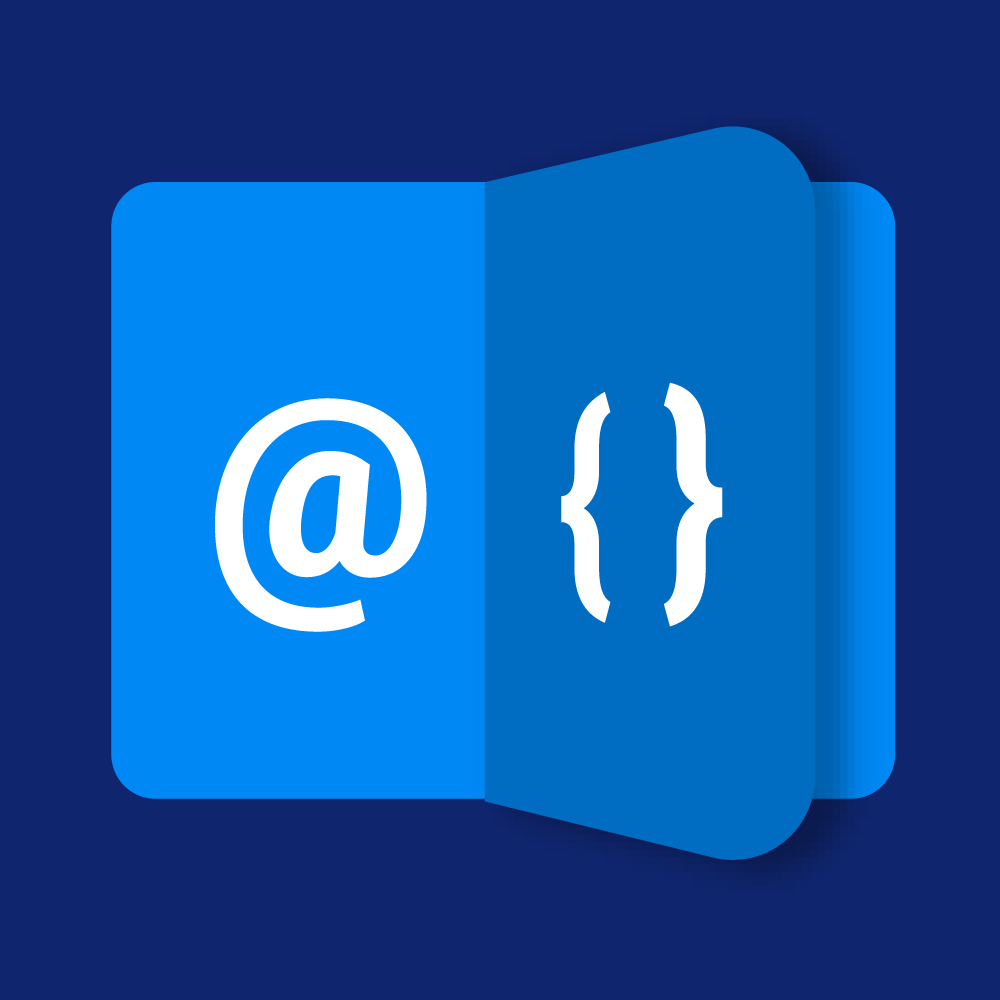
Razor Basics
Learn how to use variables and loops, encode HTML, use πππͺ Emojis. Also advanced stuff like re-using code or setting page titles and SEO stuff.
- Basic set variable and show
Create variables and show using
@variableName
- Razor Conditions such as if, if-else and ? :
if/else and ternary operator
(condition ? true : false)
- Loops -
for
andforeach
Loops using
for()
andforeach()
- Work with HTML Output
Learn the difference of showing variables with
@variable
and@Html.Raw(variable)
, and re-use very simple snippets - Working with π Emojis / Emoticons
Show Emojis in your output or use them for showing true/false
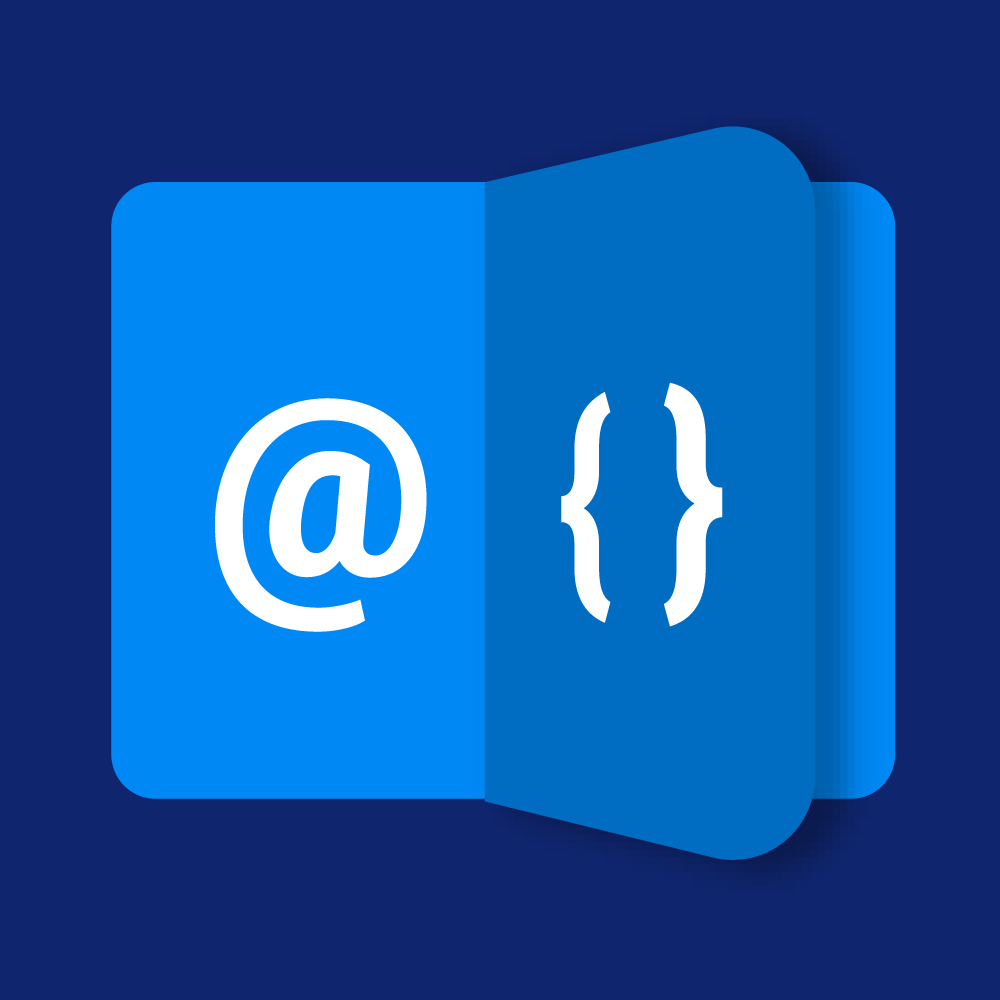
Basics: Reuse Code and Templates Across Files
You can place common templates, hlp and functions into shared files - both .cshtml
and .cs
. And you can split Razor files into code and templates.You can place common templates, hlp and functions into shared files - both .cshtml
and .cs
. And you can split Razor files into code and templates.
- Reuse Code and Razor Templates
Explains the basics of re-using code across templates and code files.
- Code - Function and Similar Helpers
Normal C# functions are the basic block for reusing code.
- Reuse Shared Templates with Html.Partial and MyModel
Reuse Razor files by calling them using
@Html.Partial
and passing parameters in DynamicModel (or MyModel). - Use Shared C# Code
Use a shared razor file to hold multiple functions / helpers, and call them one-by-one as needed.
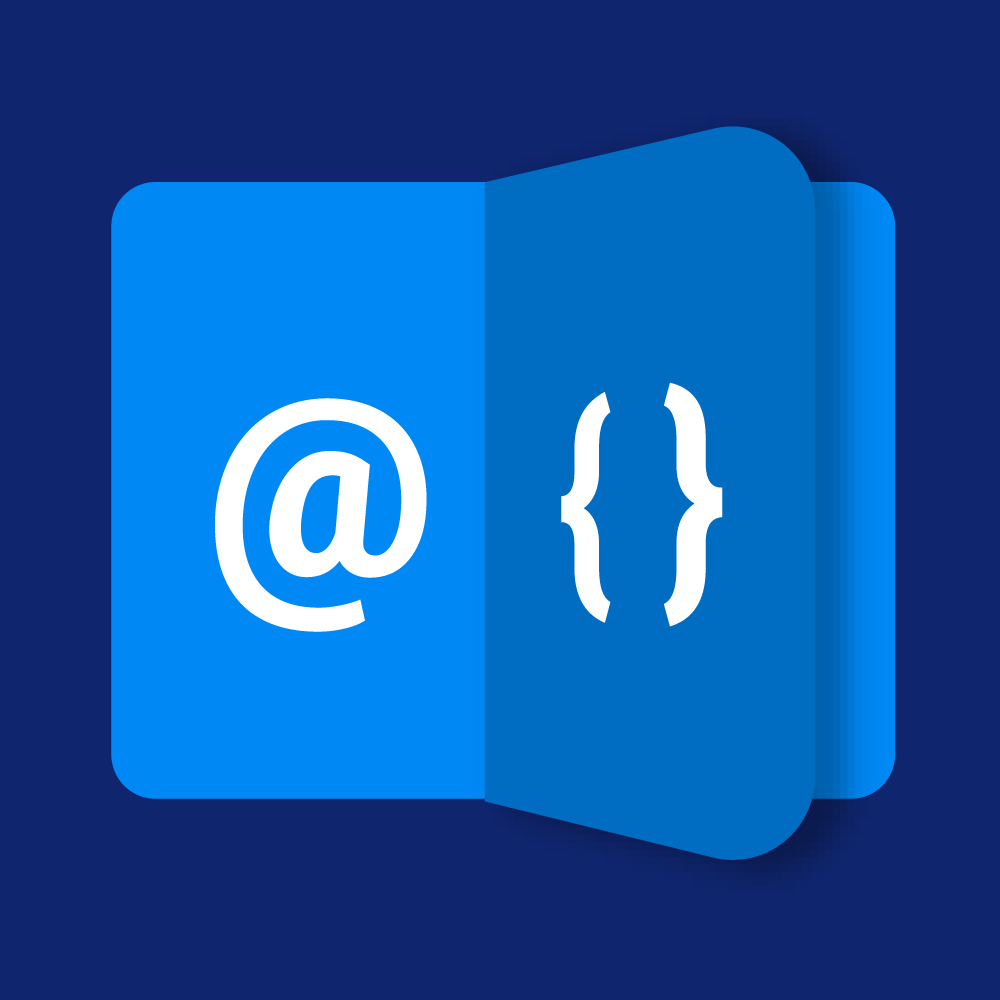
Basics: Link between Pages and Views
Learn how to use Link.To(...) to link to specific pages or to link to the same page with certain URL parameters.
- Working with Page URL Parameters
Working with text URL Parameters like ?sort=ascending
- Linking with Number Url Parameters
Working with number URL Parameters like ?id=27
- Linking with Modified Page Parameters
Learn how to create links using current URL parameters
- Switch to view by URL
Learn how to switch between views using
Link.To()
- Safely Linking URLs with Umlauts and similar
Working difficult URLs like images containing umlauts
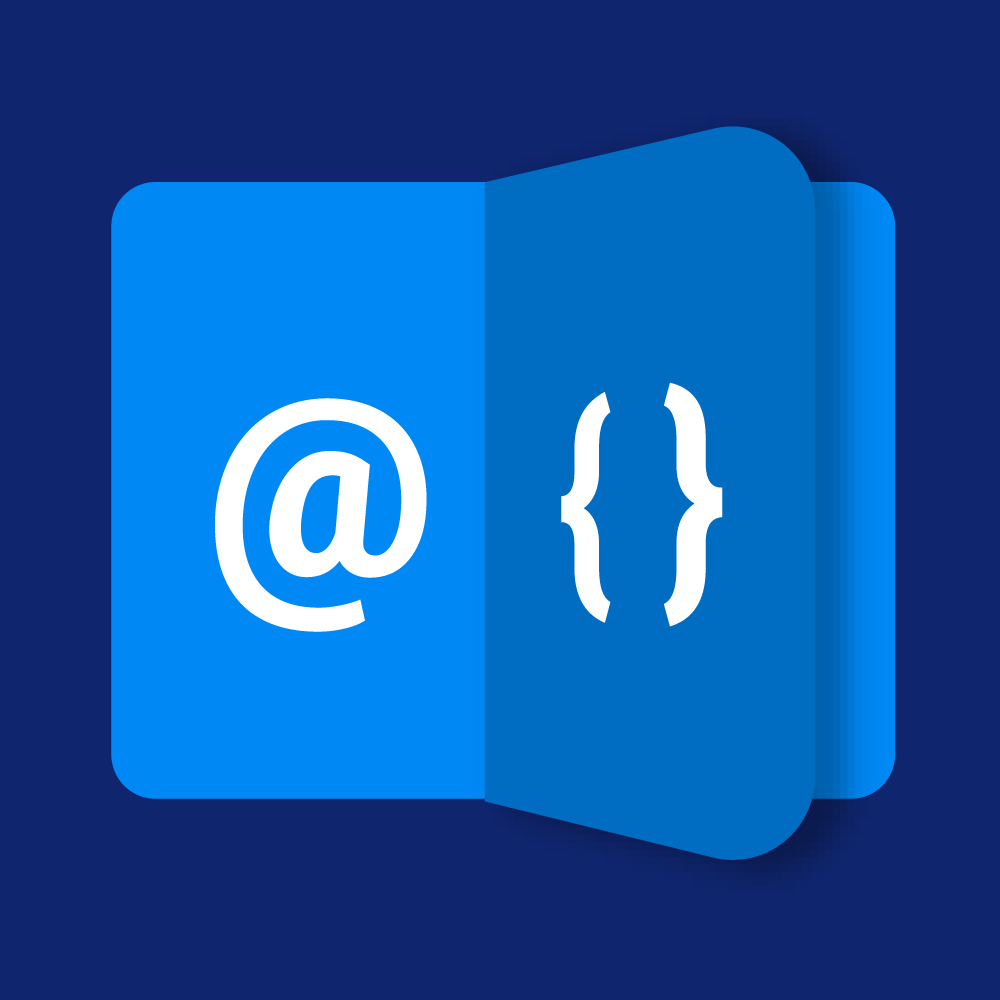
Basics: Set Page Title, Keywords etc. and Headers
Use the IPageService to set page properties, base tags and headers - even JsonLD and OpenGraph.
- Set Page Title, Keywords, Descriptions etc.
Get/Set Page Title, Keywords, Description and set meta-tags and more.
- Set <base> tag in header
Add a <base> tag to the header. This is important for SPA JS applications.
- Page Icons for Favicon, Apple/Android
Add various combinations of icons to the page header
- Meta and other Tags in header
Add all kinds of meta-tags to the header.
- JSON-LD Headers for SEO
Add JSON-LD (Linked Data) headers for Google
- Set Open-Graph headers for Social Media using the IPageService
Add Open-Graph data headers for Facebook, Twitter and other sharing-systems
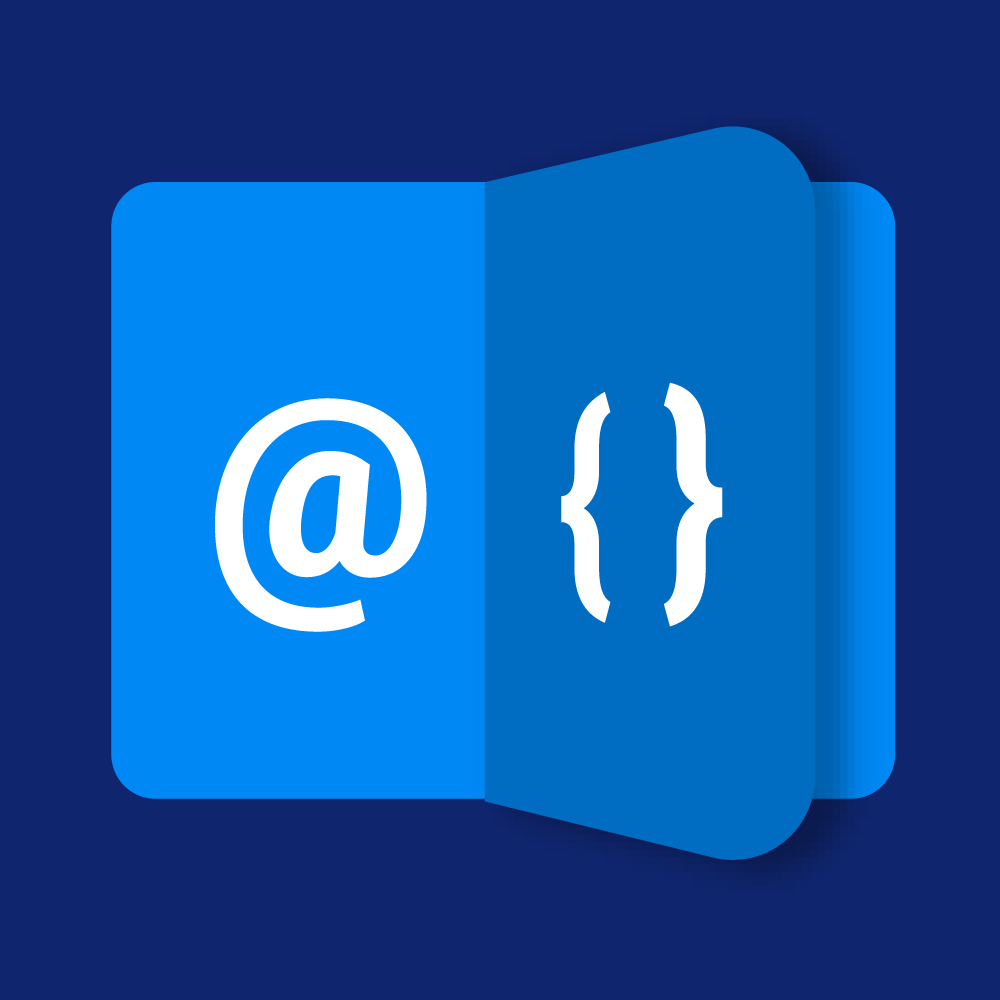
Basics: Get Information about Site, Page, User, etc.
To enable Hybrid Razor which works on Dnn and Oqtane, we need standards so that our Dynamic Code can access information about the page, module etc.
- Work with the Context (Page, Site, etc.)
Whenever you need to know about the current environment, you need Context information. These are the contexts you should know about: Platform Site Page Module View User
Content and Images
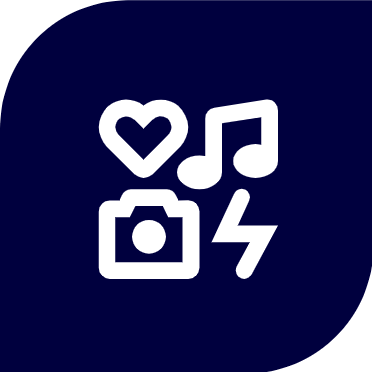
Show Content and Data Entities on a Page
- Working with Entity (Item) Values
Every thing is an Entity. Here some basic examples how to show values like Name, Birthday etc. of such an Entity.
- Working with Block Contents
Show content which was entered for this module
Images, Automatic Resizing and Responsive Pictures
Learn the basics of Images, Image Resizing and Pictures.
- The
<img>
Tag and Different Image FormatsBasics of showing images in HTML and understand JPG vs. PNG vs. WebP.
- The
Link.Image(...)
HelperLearn to use the built-in Image-Resizer to create perfect images no matter what the editor adds in the CMS.
- Resize with Reusable Settings
Store all the resize-parameters in a variable for re-use.
- Resize using Presets and Global Settings
Site-wide and App-Wide Presets help to resize with the same settings everywhere.
- Merge image settings
Merge presets with custom settings.
- Best-Possible Image for Every Screen & Device
Professional sites give each screen the best possible picture using srcset and <img>
- Browser-Capabilities
picture
with different formatsGive each screen the best possible picture based on resolution and format using
srcset
and<picture>
- Best practices using the Image Service
Learn the newest best practices using the image service
Multi-Language Content and Resources (i18n) π
- Going all Multilanguage / i18n
Everything in 2sxc can be multi-language. Discover how!
Replace Content Placeholders using TemplateService (new π)
- Template Service Tutorials new π
Learn how to use the new Template Service.
Data and DataSources
Data
Access App-Data directly or from an App-Query. Learn to query the data in C# using LINQ and work with SQL and JSON
- Working with Data
Overview about all the basics like Data vs. App.Data, Query etc.
- Basic Example from App.Data
Get any kind of data from the current App.
Data: List-Details Samples
Show data from DNN, the current App, DataSources or SQL, CSV etc. Also includes list/details (parent/child) examples.
- Basic List Details using Code
List-Details Example where a single Razor-file has the code for both the list and the details.
- Basic List Details using Code - with separate Details-File
List-Details Example where a Razor-file has the code for retrieving other Razor files for list and details.
- List Details using Automatic View-Switching
List-Details Example where the View-Configuration is setup to use a different Razor if a URL-parameter is given for the details.
- List Details using Automatic View-Switching and Query
List-Details Example where the View-Configuration is setup to use a different Razor if a URL-parameter is given for the details. In this case it will use a Query to then get the details-data.
- Look at the Content-Type of something (advanced)
Use some internal APIs to look at the definition of a Content-Type.
Data: Work with Data from Queries - Entities, SQL, CSV and more
Show data from DNN, the current App, DataSources or SQL, CSV etc. Also includes list/details (parent/child) examples.
- Example from CSV
See an example how to use a Query to get Data from a CSV file as data source.
- Various SQL Samples
Use SQL in Queries or in Code to get more data.
- Use DNN APIs to get DNN Data
DNN also provides a lot of data about users, pages etc. In this example, we'll list all the pages (tabs) in DNN and mark the one we're on. Please note that the DNN API isn't very consistent - so sometimes things use ID , sometimes Id , so best…
Data: LINQ (Language Integrated Query)
The following examples show how to use LINQ (Language INtegrated Query) to sort, filter and group data. This is for quick work in your views - for more extensive querying, we recommend the Visual Query Designer.
- LINQ Basics
Learn to use LINQ in your code.
- LINQ Relationships - Children, Parents, Grandchildren, ...
Combine LINQ with navigating Children and Parents of related data.
Data: JSON
Easily work with JSON data.
- Working with JSON Data
Easily convert JSON into usable objects in C#
DataSources: Use in C# Code
DataSources will usually be used in a VisualQuery, but you can also use them in C# for advanced scenarios. Learn about the Kit.Data API
- Data Sources Tutorial - use in C# / Razor Code
How to use DataSources directly in your Razor / C# Code.
DataSources: Create Custom Dynamic DataSources
Create custom DataSources directly in the App DataSources folder.
- Dynamic DataSources Tutorial
Learn about DataSources in general, before we start using or even creating them.
- Use Dynamic DataSources in Visual Query
Dynamic DataSources can also be used in VisualQuery.
- Custom Dynamic DataSources - Data Relationships
Create Data with Relationships to other Data - such as parent/child or tree / folder structures
Advanced Settings and Automation
- Settings in 2sxc
Settings allow your code to use predefined settings across all apps and sites. And they allow you to overide a setting at any level of the application. You can read more about the settings in the docs π . - Use PageService.Activate Features like JS/CSS
2sxc 12.04 helps with many advanced challenges like these: Make sure that certain scripts are only loaded once (like jQuery or FancyBox) Make sure that all apps use the same version of these scripts Starting things in the right order
RazorBlade and Koi
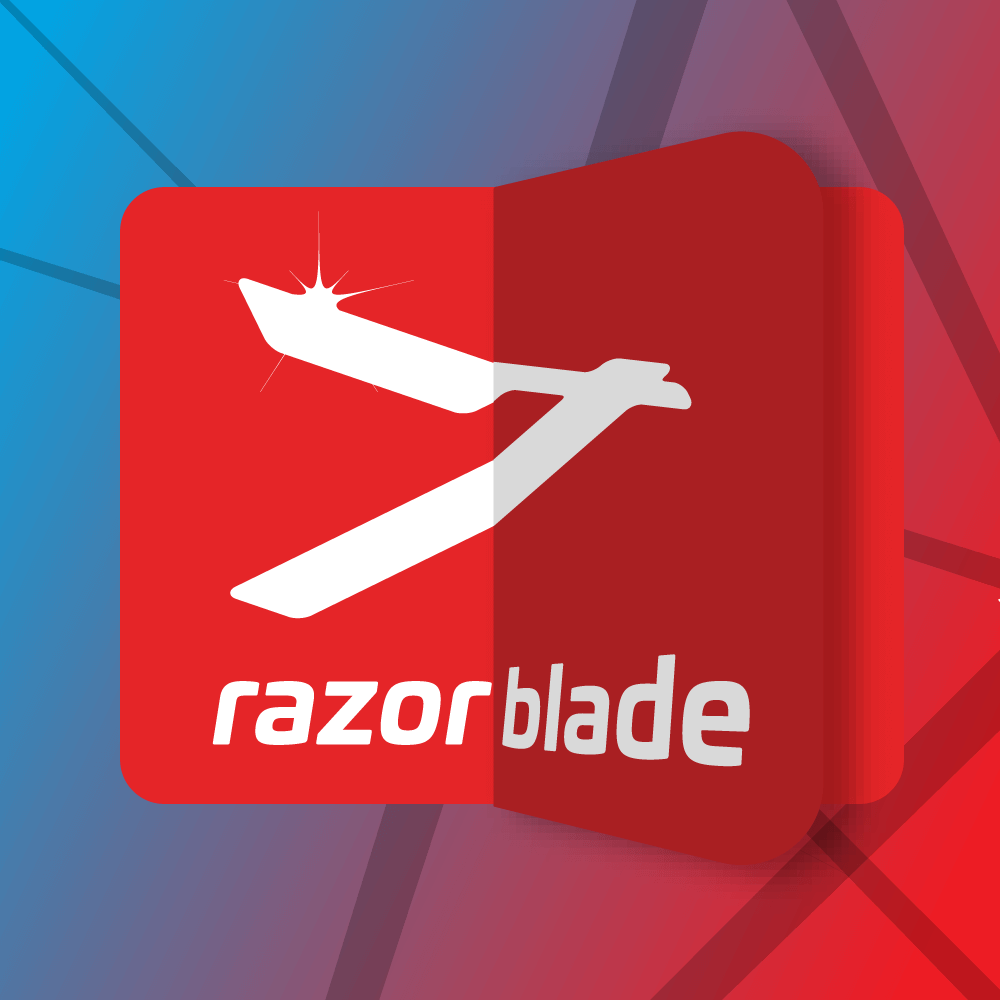
RazorBlade Tutorials
These examples help you quickly do hard stuff, once you've mastered the basics above
- RazorBlade Tutorials - Introduction
Properly create preview-texts, strip HTML, pick the right variables, set page titles and way more.
- RazorBlade Text Operations
RazorBlade helps with many helper functions to optimize working with string variables, also when they contain html. - Scrub HTML Strings (Remove HTML Tags)
These demos show how to strip all html from a string. You often need this in combination with crop or ellipsis to create teasers.
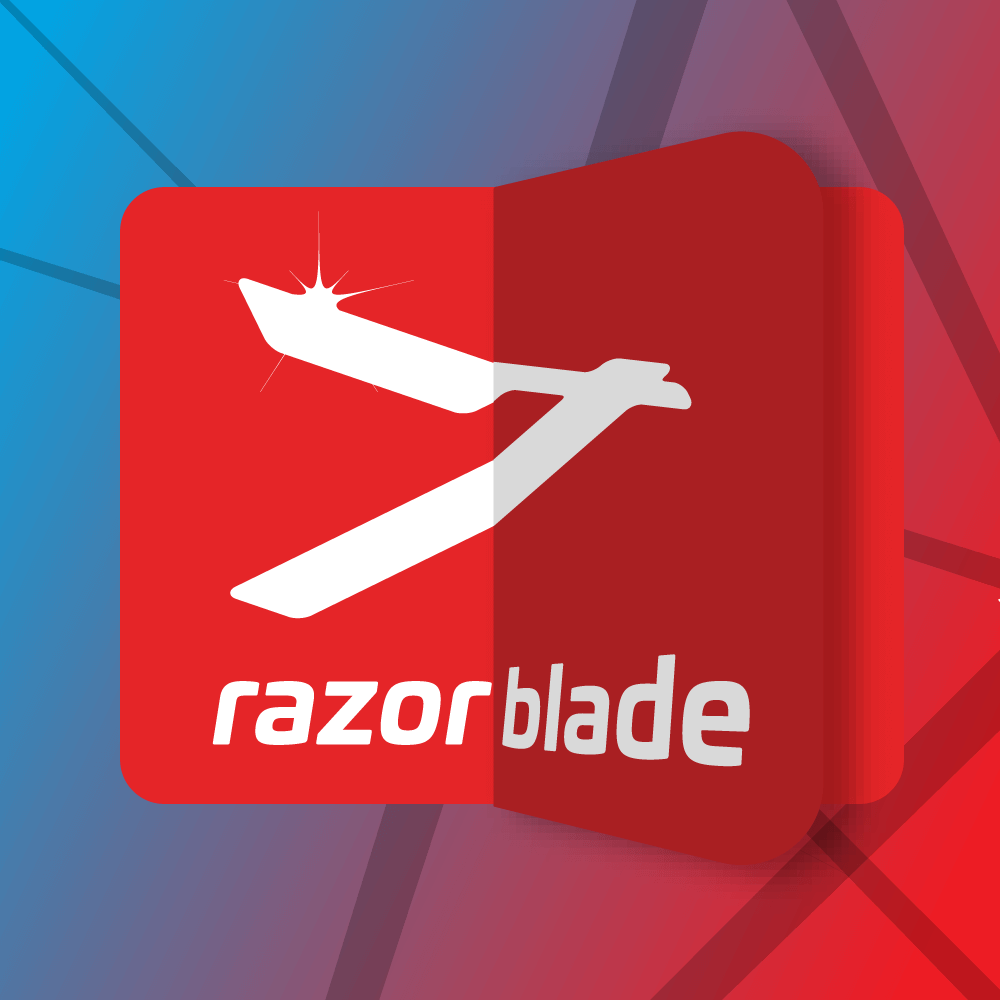
RazorBlade Fluent Html5 API
- RazorBlade Basic Html5
Tag
API Introduction v3.0The basics of creating html directly from code
- RazorBlade Fluent Tag API
@Tag.Img().Src(...)
andSrcset(...)
v3The API automatically fixes unsafe URLs like Umlauts, japanese characters etc. - SuperSmart π
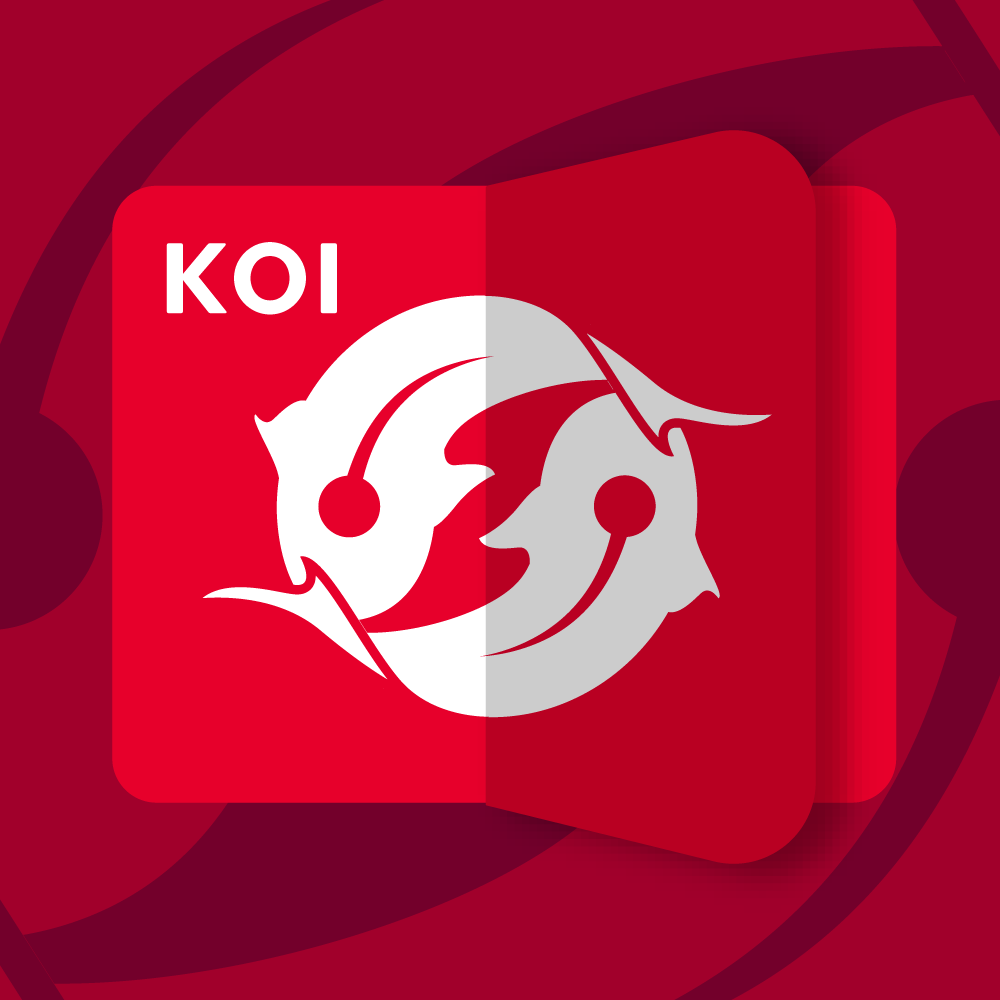
Koi Tutorials
- Use Koi to Adjust to the Page CSS Framework
Learn how to use Connect.Koi to ensure your module/template has the right CSS frameworks, to either auto-include otherwise, show messages to the admin or generate different output. This uses Connect.Koi . Some notes before we start Koi works like…
JavaScript, turnOn, WebAPIs / REST
JavaScript
- Use the 2sxc JS API to get Data
Use the 2sxc data API and get data of a specific Content-Type
- Use the sxc data API to create/edit/delete data
Use the 2sxc data API and create, update, delete data
- Use the sxc data API to create metadata
Use the 2sxc data API and create new metadata
turnOn
- turnOn Tutorial
Learn how to execute JavaScript functions using the 2sxc turnOn. Extend basic usage by passing data, awaiting conditions and more.
WebApi / JSON Endpoints Examples
- WebAPI and REST - Introduction
Provide data to SPAs and do things like sending mails, changing data and more.
- WebAPI Basics: Call WebAPIs and REST using JavaScript
Creating the simplest possible hello WebApi and a Square(number) Api.
- WebApi with Polymorph Editions
Basic example showing the same API in a live and staging editior, so you can develop while the users still use the sable one.
- App.Data WebApi Examples
Basic example reading App Data.
Edit UI and Formulas
Customize Edit UI / UX - Toolbars
Sometimes you want to customize what the editor will experience - using special toolbars or custom input fields. Note that this only applies to 2sxc.
- 2sxc Toolbar Customization
Since you can do so many things customizing the toolbar, we created a bunch of small examples for you. Note that we are basically providing a list of commands how the toolbar should be modified. - Advanced Toolbar Customizations
Customize Edit UI - Fields
Create custom input fields and customize WYSIWYG to your needs.
- 2sxc Custom Input Fields (11.02+)
2sxc 11 makes it very easy to create custom input fields using standard WebComponents . This example shows the most basic case - just a dummy message (so not a real input field). You can use it to learn about things like: Naming conventions for…
Customize Edit-UI - Formulas (new!)
Add custom UI logic to your edit forms with formulas.
- Get Started with Formulas
Create Your First Formulas, affect Values and more.
- Formulas for Pickers / DropDowns
Change what options are available in a DropDown
- Formulas affecting Field Settings
Formulas affecting Settings such as Required, Collapsed, Disabled, etc.
- Formulas affecting Groups of Fields
Modify Groups - auto-collapse based on rules, change help texts etc.
- Formulas using Parameters new v16
Create formulas which modify the form using parameters or App Settings (new v16)
- Advanced Formula APIs new v16
Do advanced things such as checking features or the current user.
Advanced / Other
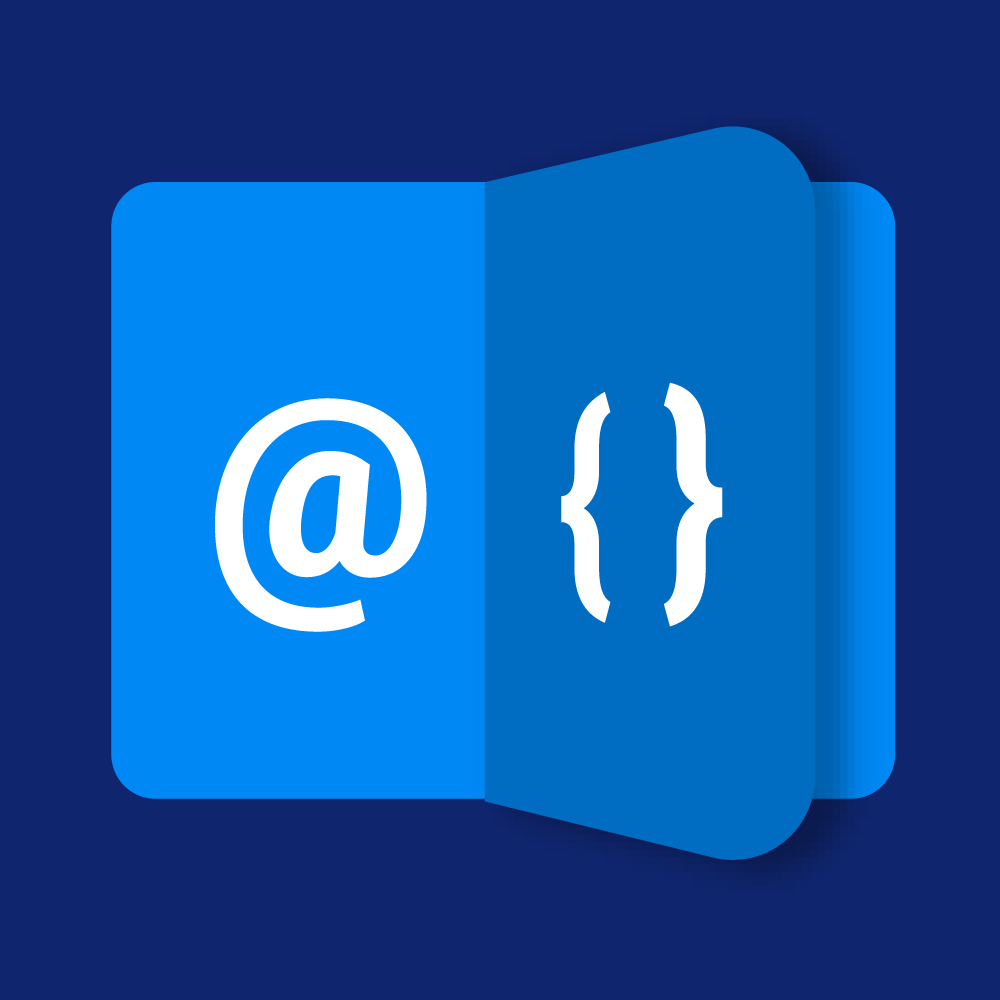
Hybrid - Advanced Scenarios for Razor on Dnn AND Oqtane
Most Razor is identical for DNN and Oqtane, but in some cases you wish to access platform specific objects or use certain features which require different code in Dnn and Oqtane. Here's how to switch between such code.
- Hybrid Razor - Code which Runs on Dnn and Oqtane
Detect the platform in various ways and adjust what your code does.
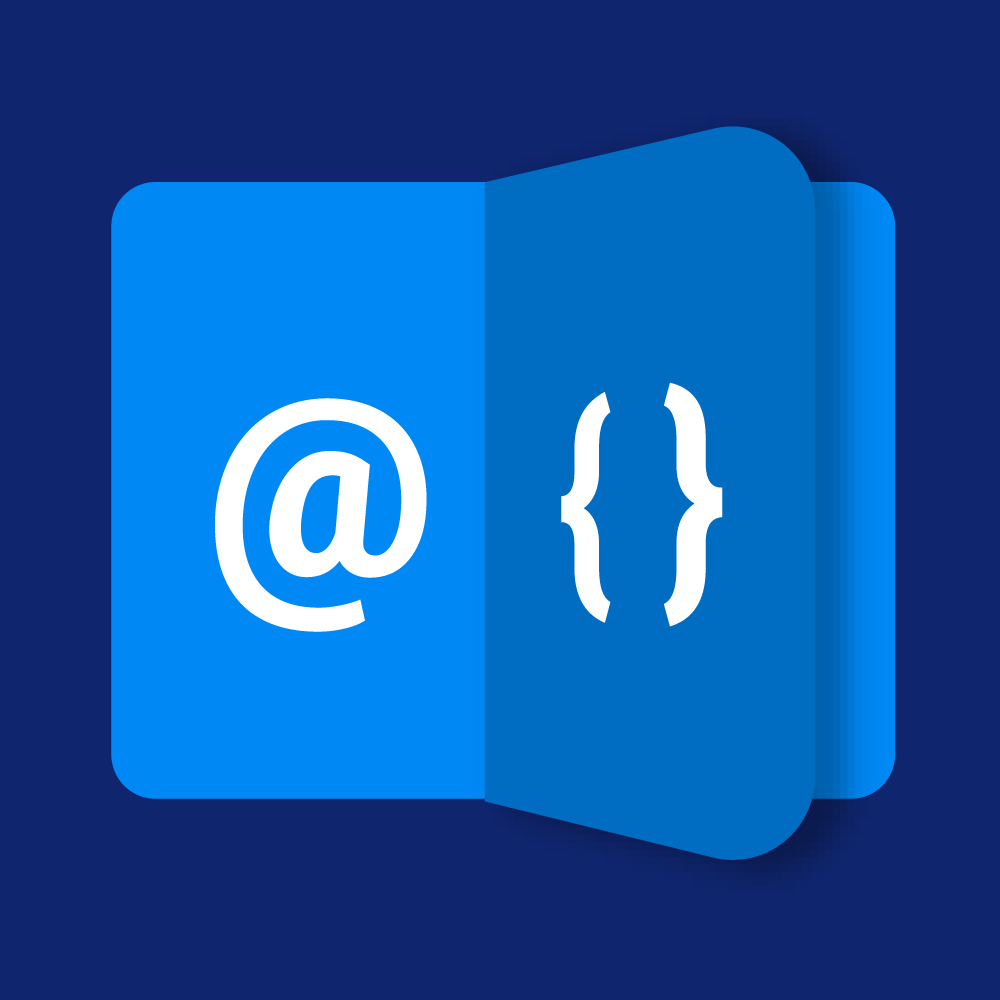
Crazy 2sxc Advanced Examples
- Inspect a Content-Block
In this example, we'll see what content-blocks are in the system, and where they are in use.
Future Tutorials (Work-In-Progress)
- Linking around in dnn
- Navigation links
- Permission examples
- Work with icon-fonts, font-awesome etc.
- Using data from other apps
- Customizing Search Results
- Customizing search from data